- In Qt, we have an alternative to the callback technique: We use signals and slots. A signal is emitted when a particular event occurs. Qt's widgets have many predefined signals, but we can always subclass widgets to add our own signals to them. A slot is a function that is called in response to a particular signal.
- Signals and Slots. In Qt, we have an alternative to the callback technique: We use signals and slots. A signal is emitted when a particular event occurs. Qt's widgets have many predefined signals, but we can always subclass widgets to add our own signals to them. A slot is a function that is called in response to a particular signal.
One key and distinctive feature of Qt framework is the use of signals and slots to connect widgets and related actions. But as powerful the feature is, it may look compelling to a lot of developers not used to such a model, and it may take some time at the beginning to get used to understand how to use signals and slots properly. However, since version 4.4, we can relay on
@user2448027 answer is correct, but there is a missing point in Qt's design pattern: different applications of private slots vs public slots. By making slot private you force users of the object to use connect function to call the slot, rather than member access operators(.
auto-connections to simplify using this feature.
Back in the old days, signals and slots connections were set up for compile time (or even run time) manually, where developers used the following sentence:
this is, we stated the sender object's name, the signal we want to connect, the receiver object's name and the slot to connect the signal to.
Now there's an automatic way to connect signals and slots by means of QMetaObject's ability to make connections between signals and
suitably-named slots. And that's the key: if we use an appropriate naming convention, signals and slots will be properly connected without the need to write additional code for that to happen. So by declaring and implementing a slot with a name that follows the following convention:
uic
(the User Interface Compiler of Qt) will automatically generate code in the dialog's
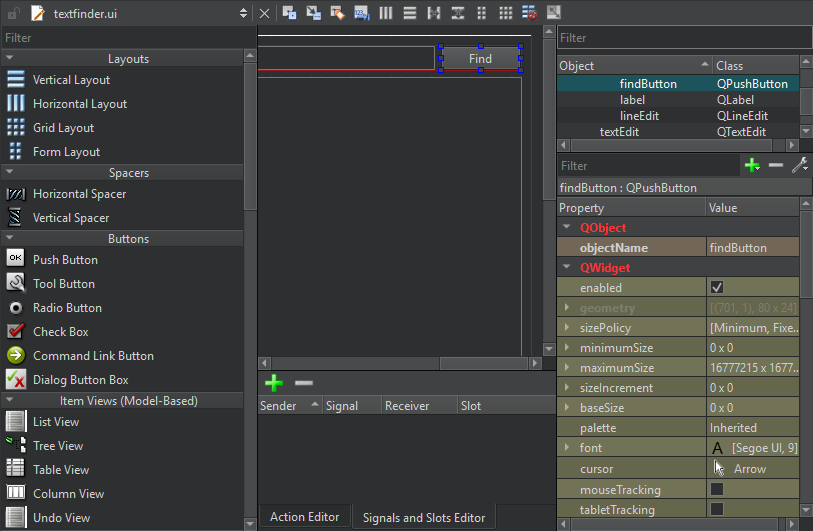
setupUi() function to connect button's signal with dialog's slot.
So back to our example, the class implementing the slot must define it like this:
Qt Private Slot Examples
We then write the method's implementatio to carry on an action when the signal is emitted:
Qt Private Slot Example Software
Qt Private Slot Example Value
In brief, we have seen that by using automatic connection of signals and slots we can count on both a standard naming convention and at the same time an explicit interface for designers to embrace. If the proper source code implements such a given interface, interface designers can later check that everything is working fine without the need to code.